Ansible in Python. In the following tutorial, we will understand Ansible along with its benefits and how we can use Ansible in Python. An Introduction to Ansible. Ansible is an open-source platform or automation tool utilized to perform IT tasks like deploying applications, managing configurations, orchestrating intra-service, and provisioning. Sep 24, 2021 Python API. This API is intended for internal Ansible use. Ansible may make changes to this API at any time that could break backward compatibility with older versions of the API. Because of this, external use is not supported by Ansible. If you want to use Python API only for executing playbooks or modules, consider ansible-runner first.
In this post, we will learn how to run a single Ansible automation task using an ad hoc command and explain some use cases for ad hoc commands.
Running ad hoc Commands with Ansible
An ad hoc command is a way of executing a single Ansible task quickly, one that you do not need to save to run again later. They are simple, online operations that can be run without writing a playbook. Ad hoc commands are useful for quick tests and changes. For example, you can use an ad hoc command to make sure that a certain line exists in the /etc/hosts file on a group of servers. You could use another ad hoc command to efficiently restart a service on many different machines or to ensure that a particular software package is up-to-date.
Ad hoc commands are very useful for quickly performing simple tasks with Ansible. They do have their limits, and in general, you will want to use Ansible Playbooks to realize the full power of Ansible. In many situations, however, ad hoc commands are exactly the tool you need to perform simple tasks quickly.
Running Ad Hoc Commands
Use the ansible command to run ad hoc commands:
The host-pattern argument is used to specify the managed hosts on which the ad hoc command should be run. It could be a specific managed host or host group in the inventory. You have already seen this used in conjunction with the –list-hosts option, which shows you which hosts are matched by a particular host pattern. You have also already seen that you can use the -i option to specify a different inventory location to use than the default in the current Ansible configuration file.
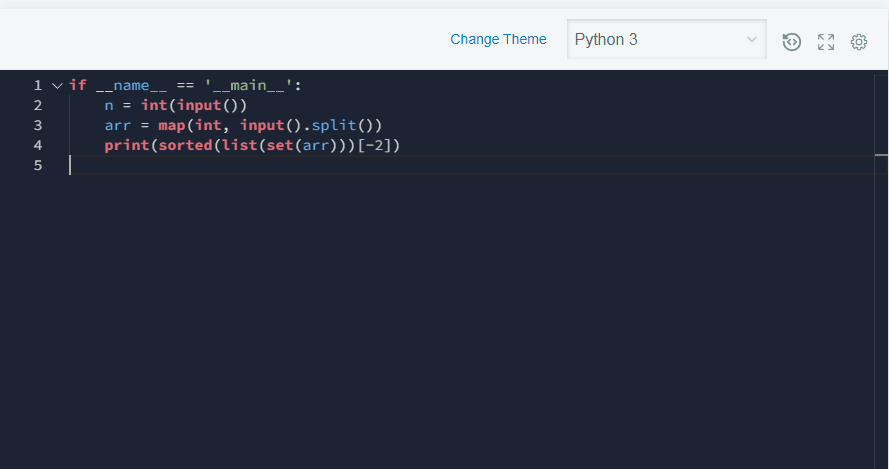
The -m option takes as an argument the name of the module that Ansible should run on the targeted hosts. Modules are small programs that are executed to implement your task. Some modules need no additional information, but others need additional arguments to specify the details of their operation. The -a option takes a list of those arguments as a quoted string.
One of the simplest ad hoc commands uses the ping module. This module does not do an ICMP ping, but checks to see if you can run Python-based modules on managed hosts. For example, the following ad hoc command determines whether all managed hosts in the inventory can run standard modules:
Performing Tasks with Modules Using Ad Hoc Commands
Modules are the tools that ad hoc commands use to accomplish tasks. Ansible provides hundreds of modules that do different things. You can usually find a tested, special-purpose module that does what you need as part of the standard installation. The ansible-doc -l command lists all modules installed on a system. You can use ansible- doc to view the documentation of particular modules by name, and find information about what arguments the modules take as options. For example, the following command displays documentation for the ping module:
The following is a list of useful modules as examples. Many others exist.
Files modules
- copy: Copy a local file to the managed host.
- file: Set permissions and other properties of files.
- lineinfile: Ensure a particular line is or is not in a file.
- synchronize: Synchronize content using rsync.
Software package modules
- package: Manage packages using autodetected package manager native to the operating system.
- yum: Manage packages using the YUM package manager.
- apt: Manage packages using the APT package manager.
- dnf: Manage packages using the DNF package manager.
- gem: Manage Ruby gems.
- pip: Manage Python packages from PyPI.
System modules
- firewalld: Manage arbitrary ports and services using firewalld.
- reboot: Reboot a machine.
- service: Manage services.
- user: Add, remove, and manage user accounts.
Net Tools modules
nmcli: Manage networking.
Most modules take arguments. You can find the list of arguments available for a module in the module’s documentation. Ad hoc commands pass arguments to modules using the -a option. When no argument is needed, omit the -a option from the ad hoc command. If multiple arguments need to be specified, supply them as a quoted space-separated list. For example, the following ad hoc command uses the user module to ensure that the newbie user exists and has UID 4000 on servera.lab.example.com:
Most modules are idempotent, which means that they can be run safely multiple times, and if the system is already in the correct state, they do nothing. For example, if you run the previous ad hoc command again, it should report no change:
Running Arbitrary Commands on Managed Hosts
The command module allows administrators to run arbitrary commands on the command line of managed hosts. The command to be run is specified as an argument to the module using the -a option. For example, the following command runs the hostname command on the managed hosts referenced by the mymanagedhosts host pattern.
The previous ad hoc command example returned two lines of output for each managed host. The first line is a status report, showing the name of the managed host that the ad hoc operation ran on, as well as the outcome of the operation. The second line is the output of the command executed remotely using the Ansible command module.
For better readability and parsing of ad hoc command output, administrators might find it useful to have a single line of output for each operation performed on a managed host. Use the -o option to display the output of Ansible ad hoc commands in a single line format.
The command module allows administrators to quickly execute remote commands on managed hosts. These commands are not processed by the shell on the managed hosts. As such, they cannot access shell environment variables or perform shell operations, such as redirection and piping.
For situations where commands require shell processing, administrators can use the shell module. Like the command module, you pass the commands to be executed as arguments to the module in an ad hoc command. Ansible then executes the command remotely on the managed hosts. Unlike the command module, the commands are processed through a shell on the managed hosts. Therefore, shell environment variables are accessible and shell operations such as redirection and piping are also available for use.
The following example illustrates the difference between the command and shell modules. If you try to execute the built-in Bash command set with these two modules, it only succeeds with the shell module.
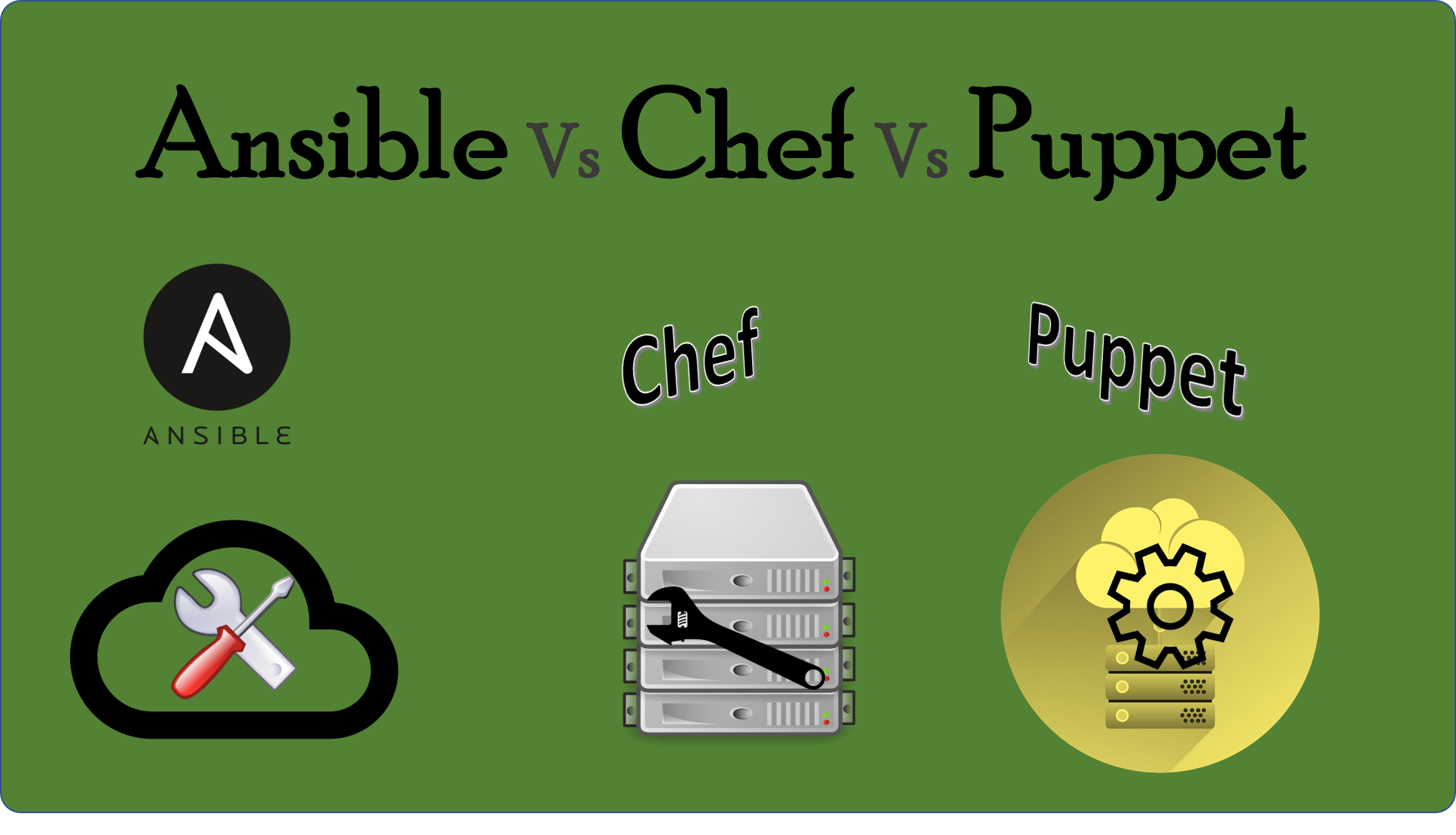
Both command and shell modules require a working Python installation on the managed host. A third module, raw, can run commands directly using the remote shell, bypassing the module subsystem. This is useful when managing systems that cannot have Python installed (for example, a network router). It can also be used to install Python on a host.
Configuring Connections for Ad Hoc Commands
The directives for managed host connections and privilege escalation can be configured in the Ansible configuration file, and they can also be defined using options in ad hoc commands. When defined using options in ad hoc commands, they take precedence over the directive configured in the Ansible configuration file. The following table shows the analogous command-line options for each configuration file directive.
CONFIGURATION FILE DIRECTIVES | COMMAND-LINE OPTION |
---|---|
inventory | -i |
remote_user | -u |
become | –become, -b |
become_method | –become-method |
become_user | –become-user |
become_ask_pass | –ask-become-pass, -K |
Before configuring these directives using command-line options, their currently defined values can be determined by consulting the output of ansible –help.
What are cron jobs?
In many UNIX/LINUX type operating systems, the Cron is one of the very valuable and productive utility. It is used to run commands and scripts at pre-defined time.
These pre-scheduled jobs are called 'cron jobs'.
Some Common Cron Jobs Use Cases
- Deleting Old/Unused Files
- Regular Checking of Disk Space
- Regular Pre-Scheduled Backups
- Operating System Maintenance Jobs
- etc.
How to create a simple cron job? (Without Ansible)
Run the follwoing command at linux shell prompt:
- linux-prompt$ crontab -l # To list the crontab enteries
- linux-prompt$ crontab -e # To edit/add crontab enteries
Following is the syntax of cron job entry in the crontab file:
For Example:
- To run /path/command ten minutes every day, after midnight, following will be the entry:
10 0 * * * /path/command
- To run /path/myscript.sh at 3:30 PM on the fifth day of every month, following will be the entry:
30 15 5 * * /path/myscript.sh
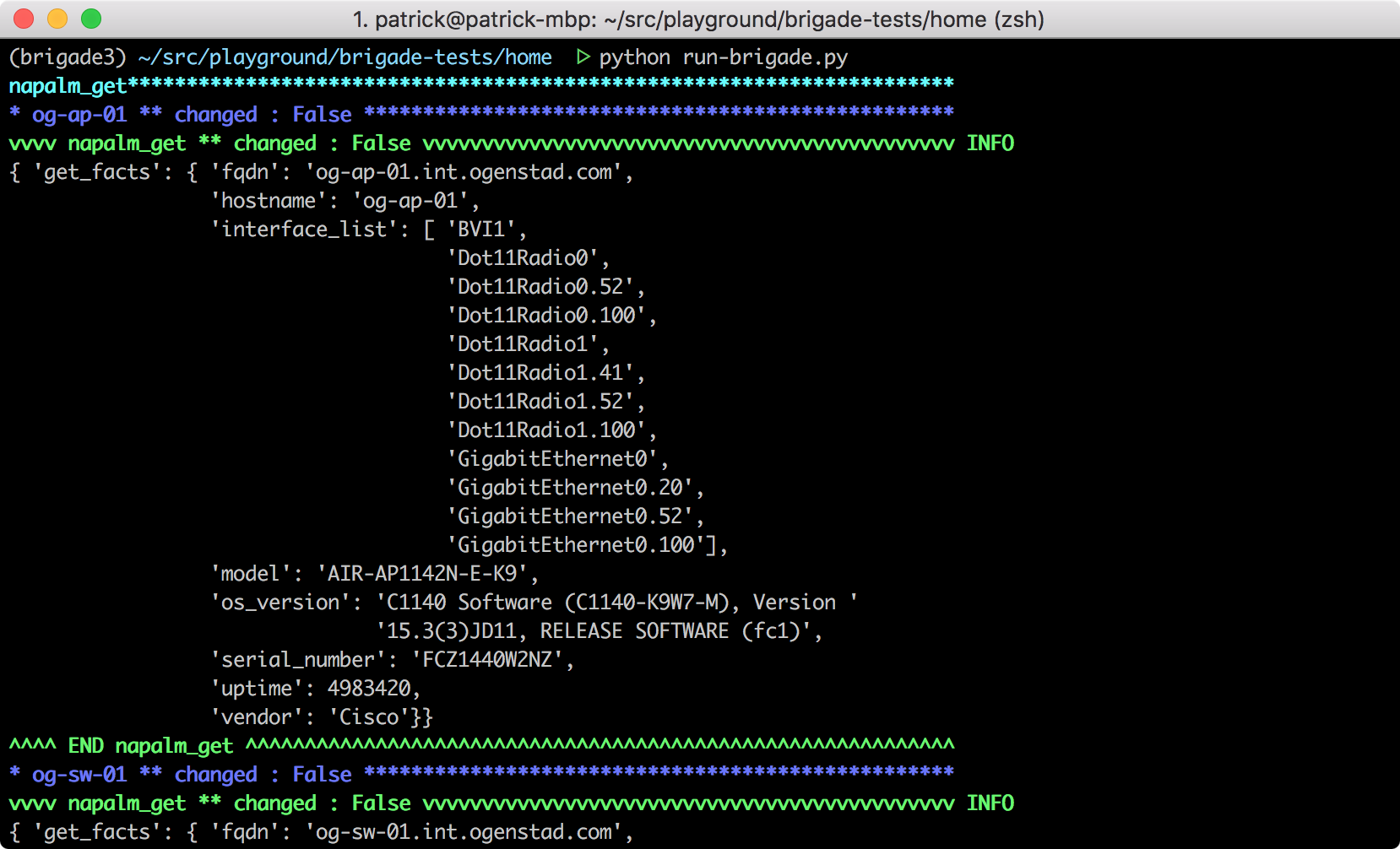
- To run /path/myscript.sh at 6 PM on selected working weekdays (Monday, Tuesday and Wednesday), following will be the entry:
0 18 * * 1-3 /path/myscript.sh

How Ansible is useful to manage cron jobs?
The Ansible cron module is used to manage the crontab entries via play books. Consider, If you have many servers and you want to configure some common cron jobs on all servers, then configuring the same job manually on all servers will be tedious and error-prone task. Ansible can do this job via a simple playbook to configure the said cron jobs on all servers automatically (in one go).
Step by Step Example of using Ansible CRON module
Pre-Requisites:
1- One Ansible Control Node
Running Ansible From Python
2- Two Ansible managed hosts (You may use as many as you want)
3- Network access between control node and managed nodes
4- Host names of all three nodes should be registered with DNS server or appropriate entries should be present in the /etc/hosts files (on all three nodes).
5- User SSH keys should have already been generated at control node and shared with managed nodes (see this article to configure SSH Keys: http://tiny.cc/ro75fz
Note: In this article, we have used one user 'fakhar' on all three nodes. Its SSH keys have been generated at control node and shared on both managed hosts.
Step-1: Create an inventory file at control node (i.e. CentOS-Ctrl-Node), containing the host names of both managed servers, as shown in below image:
Step-2: Create an ansible playbook, to schedule cron jobs as shown below:
Ansible Runner Python Example Code
Step-3 (Optional): Run the 'tree' command at control node, where your playbook is placed (this is just to show you as how the files are placed/organized). See image below:
Step-4: Run the 'crontab -l' and 'ls -l /home/fakhar/temp.txt' on both managed hosts to confirm the status before the execution of playbook. See image below:
Step-5: Run the playbook using command 'ansible-playbook -i inventory cronPlayBook.yml' at control node. See image below. The playbook has been executed successfully.
Step-6: Check the result on the target managed hosts after the cron job scheduled time. For this purpose, run the 'crontab -l' and 'ls -l /home/fakhar/temp/txt' commands on both managed hosts. See image below, the cron job entries have been created and executed at specified time. The cron jobs have collected the system information and placed it in the target file (i.e. /home/fakhar/temp.txt) successfully.
Thank you.